Generate Random Api Key Javascript
- Generate Random Api Key Javascript Download
- Javascript Generate Key
- Generate Random Api Key Javascript Pdf
- Mar 28, 2012 I'm writing this in the hopes that others may find this on Google. I had an issue that I'm using a v-for in Vue.js on a component, but in Vue 2.2+ you required:key to be set with a unique identifier. In my case the Array that it iterates over doesn't have any, so I had to generate one on demand (imagine a Bootstrap alerts manager component, e.g.).
- In RandomAPI, all of your fields are attached to the api object. A field is a Javascript property that holds the data that you want to generate and the api object is the central variable that RandomAPI will end up pushing into a results array. API Codeapi.age = random.numeric(1, 25); api.dogYears = api.age. 7.
- Extractable is a Boolean indicating whether it will be possible to export the key using SubtleCrypto.exportKey or SubtleCrypto.wrapKey. KeyUsages is an Array indicating what can be done with the newly generated key.
APIs are coded in Javascript and are very easy to make! An easy to use implementation, clean interface, and RESTful API make RandomAPI simple to use in all your applications. 'User 1', 'User 2', etc., you can generate random data that is custom tailored to your app that gives it a more realistic preview to how your product will look in. JavaScript exercises, practice and solution: Write a JavaScript program to display a random image (clicking on a button) from the following list.
Nintendo wii u master key generator. Secure context
This feature is available only in secure contexts (HTTPS), in some or all supporting browsers.
Use the generateKey()
method of the SubtleCrypto
interface to generate a new key (for symmetric algorithms) or key pair (for public-key algorithms).
Syntax
Generate Random Api Key Javascript Download
Parameters
algorithm
is a dictionary object defining the type of key to generate and providing extra algorithm-specific parameters.- For RSASSA-PKCS1-v1_5, RSA-PSS, or RSA-OAEP: pass an
RsaHashedKeyGenParams
object. - For ECDSA or ECDH: pass anÂ
EcKeyGenParams
object. - For HMAC: pass an
HmacKeyGenParams
object. - For AES-CTR, AES-CBC, AES-GCM, or AES-KW: pass an
AesKeyGenParams
object.
- For RSASSA-PKCS1-v1_5, RSA-PSS, or RSA-OAEP: pass an
extractable
is aBoolean
indicating whether it will be possible to export the key usingSubtleCrypto.exportKey()
orSubtleCrypto.wrapKey()
.keyUsages
 is anArray
indicating what can be done with the newly generated key. Possible values for array elements are:encrypt
: The key may be used toencrypt
messages.decrypt
: The key may be used todecrypt
messages.sign
: The key may be used tosign
messages.verify
: The key may be used toverify
signatures.deriveKey
: The key may be used inderiving a new key
.deriveBits
: The key may be used inderiving bits
.wrapKey
: The key may be used towrap a key
.unwrapKey
: The key may be used tounwrap a key
.
Return value

result
is aPromise
that fulfills with aCryptoKey
(for symmetric algorithms) or aCryptoKeyPair
(for public-key algorithms).
Exceptions
The promise is rejected when the following exception is encountered:
SyntaxError
- Raised when the result is a
CryptoKey
of typesecret
orprivate
butkeyUsages
is empty. SyntaxError
- Raised when the result is a
CryptoKeyPair
and itsprivateKey.usages
attribute is empty.
Examples
RSA key pair generation
This code generates an RSA-OAEP encryption key pair. See the complete code on GitHub.
Elliptic curve key pair generation
This code generates an ECDSA signing key pair. See the complete code on GitHub.
HMAC key generation
This code generates an HMAC signing key. See the complete code on GitHub.
AES key generation
This code generates an AES-GCM encryption key. See the complete code on GitHub.
Specifications
Specification | Status | Comment |
---|---|---|
Web Cryptography API The definition of 'SubtleCrypto.generateKey()' in that specification. | Recommendation | Initial definition. |
Browser compatibility
Desktop | Mobile | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
Chrome | Edge | Firefox | Internet Explorer | Opera | Safari | Android webview | Chrome for Android | Firefox for Android | Opera for Android | Safari on iOS | Samsung Internet | |
generateKey | ChromeFull support 37 | EdgePartial support12
| FirefoxFull support 34
| IEPartial support11 Notes
| OperaFull support 24 | SafariFull support 7 | WebView AndroidFull support 37 | Chrome AndroidFull support 37 | Firefox AndroidFull support 34
| Opera AndroidFull support 24 | Safari iOSFull support 7 | Samsung Internet AndroidFull support 6.0 |
Legend
- Full support Â
- Full support
- Partial support Â
- Partial support
- See implementation notes.
- See implementation notes.
- User must explicitly enable this feature.
- User must explicitly enable this feature.
See also
- Cryptographic key length recommendations.
- NIST cryptographic algorithm and key length recommendations.
The Crypto.getRandomValues()
method lets you get cryptographically strong random values. The array given as the parameter is filled with random numbers (random in its cryptographic meaning).
To guarantee enough performance, implementations are not using a truly random number generator, but they are using a pseudo-random number generator seeded with a value with enough entropy. The PRNG used differs from one implementation to the other but is suitable for cryptographic usages. Implementations are also required to use a seed with enough entropy, like a system-level entropy source.
getRandomValues()
 is the only member of the Crypto
 interface which can be used from an insecure context.
Syntax
Parameters
typedArray
- An integer-based
TypedArray
, that is anInt8Array
, aUint8Array
, anInt16Array
, aUint16Array
, anInt32Array
, or aUint32Array
. All elements in the array are overwritten with random numbers.
Return value
The same array passed as typedArray
 but with its contents replaced with the newly generated random numbers. Note that typedArray
is modified in-place, and no copy is made.
Exceptions
This method can throw an exception under error conditions.
QuotaExceededError
- The requested length exceeds 65,536 bytes.
Usage notes
Don't use getRandomValues()
 to generate encryption keys. Instead, use the generateKey()
method. There are a few reasons for this; for example, getRandomValues()
 is not guaranteed to be running in a secure context.
There is no minimum degree of entropy mandated by the Web Cryptography specification. User agents are instead urged to provide the best entropy they can when generating random numbers, using a well-defined, efficient pseudorandom number generator built into the user agent itself, but seeded with values taken from an external source of pseudorandom numbers, such as a platform-specific random number function, the Unix /dev/urandom
 device, or other source of random or pseudorandom data.
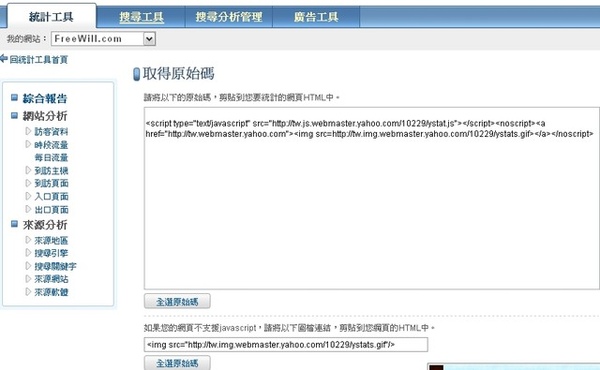
Examples
Specification
Specification | Status | Comment |
---|---|---|
Web Cryptography API | Recommendation | Initial definition |
Browser compatibility
Javascript Generate Key
Update compatibility data on GitHubDesktop | Mobile | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
Chrome | Edge | Firefox | Internet Explorer | Opera | Safari | Android webview | Chrome for Android | Firefox for Android | Opera for Android | Safari on iOS | Samsung Internet | |
getRandomValues | ChromeFull support 11 | EdgeFull support 12 | FirefoxFull support 26 | IEFull support 11 | OperaFull support 15 | SafariFull support 6.1 | WebView AndroidFull support ≤37 | Chrome AndroidFull support 18 | Firefox AndroidFull support 26 | Opera AndroidFull support 14 | Safari iOSFull support 6.1 | Samsung Internet AndroidFull support 1.0 |
Legend
- Full support Â
- Full support
See also
Generate Random Api Key Javascript Pdf
Window.crypto
to get aCrypto
object.Math.random
, a non-cryptographic source of random numbers.