Sdes P10 And P8 Key Generation
- Rotates the given number left by one. Defaults to a 5-bit word.
- def left_shift(num, length=5):
- for x inrange(length):
- Returns the bit value of the 'pos'th bit in num. Defaults to little endian positioning. (MSB is position 1)
- def get_bit(num, pos, size=8, little_endian=True):
- return(num >>(size - pos)) & 1;
- return(num >> pos) & 1;
- ''
- Permutate the input's bits according to the given permutation array.
- The permutation array describes which input bits make up the output, and where they're positioned.
- def permutate(input_num, permutation, input_size=8):
- for p inrange(len(permutation)):
- ret_num += get_bit(input_num, permutation[p], input_size)<<(len(permutation) - 1 - p);
- return ret_num;
- ''
- ''
- return permutate(num,[3,5,2,7,4,10,1,9,8,6], input_size=10);
- def P8(num):
- return permutate(num,[6,3,7,4,8,5,10,9], input_size=10);
- def P4(num):
- return permutate(num,[2,6,3,1,4,8,5,7], input_size=8);
- def IP_inverse(num):
- return permutate(num,[4,1,3,5,7,2,8,6], input_size=8);
- def E_P(num):
- return permutate(num,[4,1,2,3,2,3,4,1], input_size=4);
- ''
- Performs the S0-box calculation on the 4-bit input 'num'.
- def S0(num):
- ,(3,2,1,0)
- ,(3,1,3,2))
- row =(get_bit(num,1,4)<<1) + get_bit(num,4,4);
- Performs the S1-box calculation on the 4-bit input 'num'.
- def S1(num):
- ,(2,0,1,3)
- ,(2,1,0,3))
- row =(get_bit(num,1,4)<<1) + get_bit(num,4,4);
- Performs the switching function. Switches the left four bits of 'num' with the right four bits.
- def SW(num):
- Generates the two subkeys K1 and K2 for use in the SDES algorithm.
- ''
- key = P10(key);
- key =( left_shift(key >>5)<<5) + ( left_shift(key & 0b11111))
- K1 = P8(key);
- key =( left_shift(key >>5)<<5) + ( left_shift(key & 0b11111))
- key =( left_shift(key >>5)<<5) + ( left_shift(key & 0b11111))
- K2 = P8(key);
- return(K1, K2);
- ''
- Performs one round of the Feistel cipher on the provided number.
- def f_K(num, subkey):
- right_input = num & 0b1111;
- data = E_P(right_input);
- data =(S0(data >>4)<<2) + S1(data & 0b1111);
- left_output = left_input ^ data;
- return(left_output <<4) + right_input;
- ''
- Encrypts the provided plaintext byte using the two subkeys, K1 and K2.
- def encrypt_byte(plaintext, K1, K2):
- round= f_K(SW(round), K2);
- ciphertext = IP_inverse(round);
- return ciphertext;
- ''
- Decrypts the provided ciphertext byte using the two subkeys, K1 and K2.
- def decrypt_byte(ciphertext, K1, K2):
- round= f_K(SW(round), K1);
- plaintext = IP_inverse(round);
- return plaintext;
- ''
- Encrypt or decrypt the input using SDES in electronic code book mode.
- input_data: The data to process. Expects a byte-array.
- mode: Whether to encrypt or decrypt the data. Defaults to encryption.
- def ecb(input_data, key, mode='encrypt'):
- for b in input_data:
- processed_byte = encrypt_byte(b, K1, K2);
- processed_byte = decrypt_byte(b, K1, K2);
- # Help text if not given enough arguments.
- print('***********************');
- print('***********************n');
- print(f'Usage:ntEncryption: python3 {sys.argv[0]} -e 'key_in_binary' 'plaintext_file' 'ciphertext_file'ntDecryption: python3 {sys.argv[0]} -d 'key_in_binary' 'ciphertext_file' 'plaintext_file');
- print(f'Example: python3 {sys.argv[0]} -e 1100101010 plaintext.txt cipertext.sdes');
- withopen(sys.argv[3],'rb')as input_file:
- chunk=bytearray(input_file.read(65535));# Process the file in 64kB chunks
- key =int(sys.argv[2],2);# Grab and decode the provided cipher key
- if(sys.argv[1]'-e'): # Encrypt the data
- elif(sys.argv[1]'-d'): # Decrypt the data
- output_file.write( ecb(chunk, key, mode='decrypt'));
- # Error case if an improper mode is provided.
- sys.stderr.write(f'Mode '{sys.argv[1]}' is not supported! Please use '-e' or '-d'!n');
- chunk= input_file.read(65535);
- main()
ISE334/SE425: E-Commerce, Com., and Info. Security Recitation 3 Semester 2 5774 12 March 2014 Simpli ed DES 1 Introduction In this lab we will work through a simpli ed version of the DES algorithm. What is Simplified DES. Nintendo wii u master key generator. Developed 1996 as a teaching tool.Santa Clara University.Prof. Edward Schaefer.Takes an 8-bit block plaintext, a 10 –bit key and produces an 8-bit block of ciphertext.Decryption takes the 8-bit block of ciphertext, the same 10-bit key and produces the original 8. Symmetric key cryptography is useful if you want to encrypt files on your computer, and you intend to decrypt them yourself. It is less useful if you intend to send them to someone else to be decrypted, because in that case you have a 'key distribution problem': securely communicating the encryption key to your correspondent may not be much easier than securely communicating the original text.
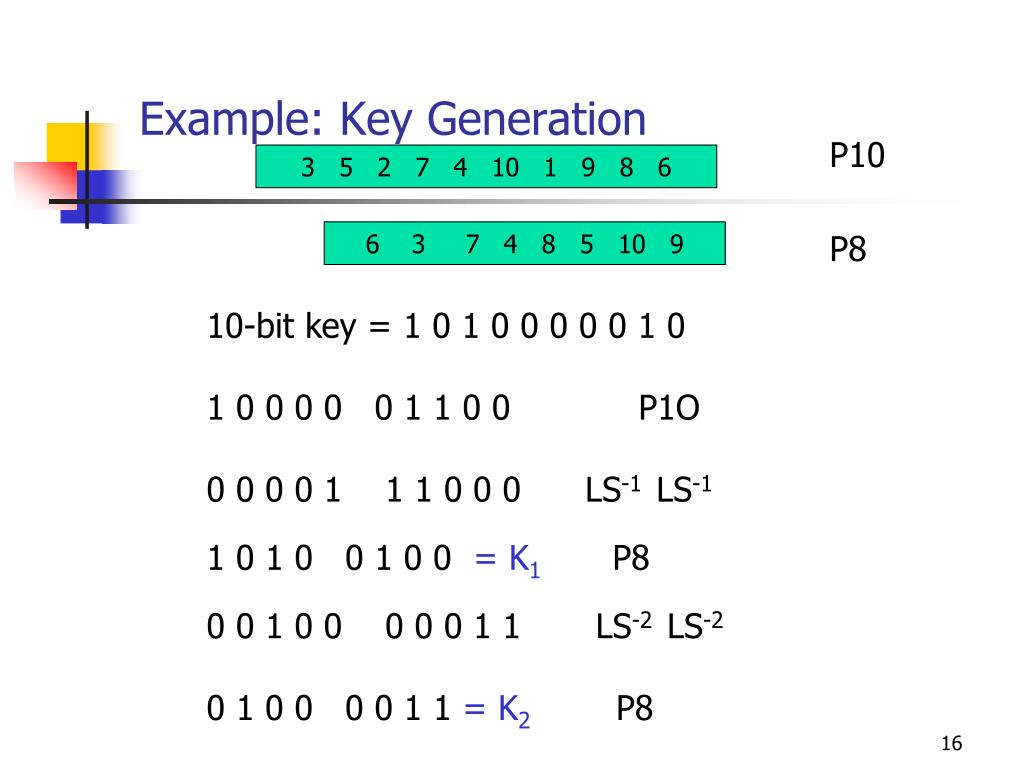
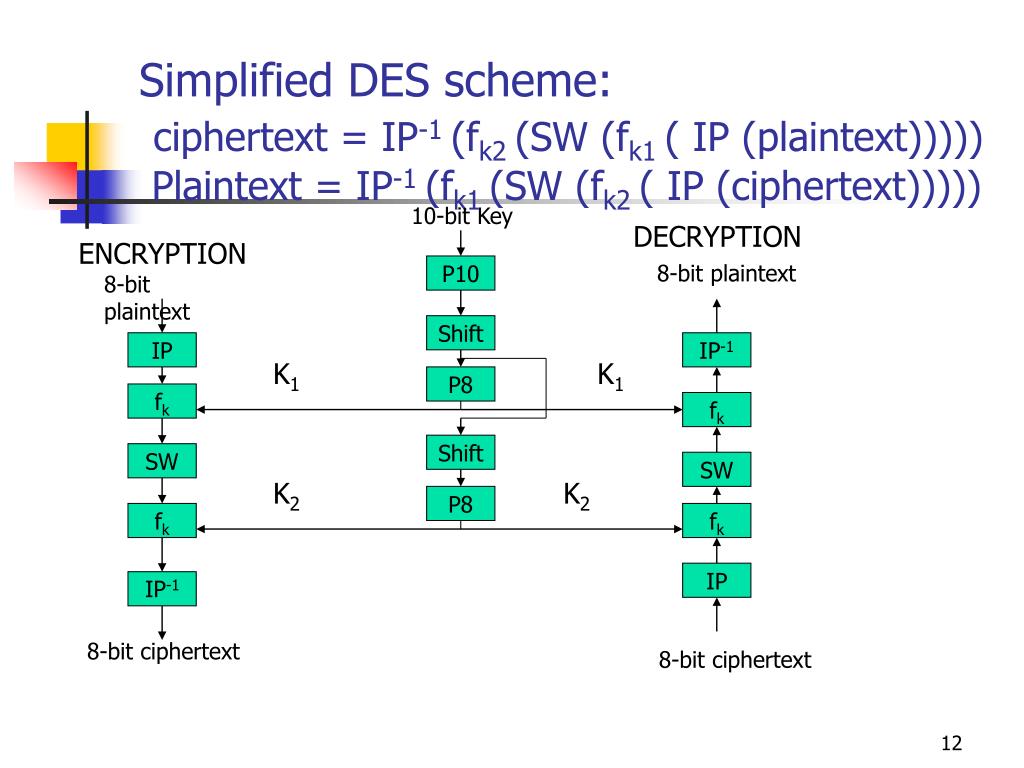
Sdes P10 And P8 Key Generation Download
It is well written overall, but remember that string += thing is very slow when used in loops and you should join a generator expression for efficiency. Share improve this answer answered Oct 19 '15 at 20:33. Nov 14, 2018 S-DES key generation.